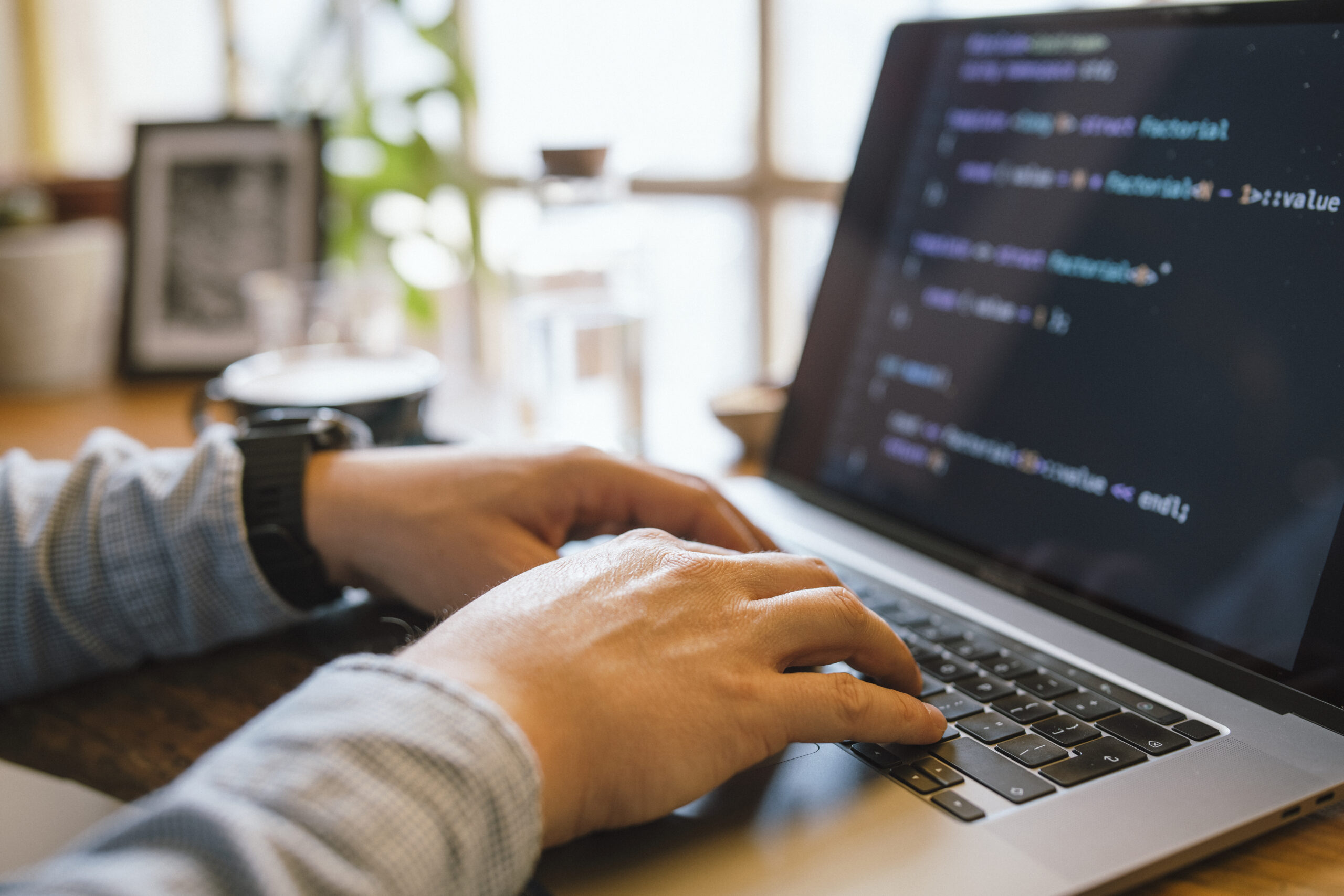
Debugging is Among the most essential — but generally missed — abilities inside a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why matters go wrong, and learning to Believe methodically to solve issues effectively. Regardless of whether you're a newbie or even a seasoned developer, sharpening your debugging expertise can preserve hours of aggravation and drastically enhance your productivity. Listed here are a number of strategies to help builders stage up their debugging match by me, Gustavo Woltmann.
Master Your Tools
One of the fastest methods developers can elevate their debugging competencies is by mastering the instruments they use every single day. Even though creating code is one Element of progress, knowing ways to communicate with it effectively all through execution is Similarly essential. Modern progress environments arrive equipped with impressive debugging capabilities — but many builders only scratch the surface area of what these applications can perform.
Consider, for example, an Built-in Improvement Ecosystem (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These tools help you set breakpoints, inspect the worth of variables at runtime, phase through code line by line, and in many cases modify code around the fly. When made use of accurately, they let you observe exactly how your code behaves for the duration of execution, which is priceless for tracking down elusive bugs.
Browser developer equipment, such as Chrome DevTools, are indispensable for front-close developers. They help you inspect the DOM, check community requests, view true-time performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can convert aggravating UI difficulties into manageable duties.
For backend or process-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage in excess of functioning processes and memory management. Finding out these applications might have a steeper Finding out curve but pays off when debugging general performance issues, memory leaks, or segmentation faults.
Past your IDE or debugger, become cozy with Model Regulate units like Git to know code historical past, uncover the precise minute bugs were being released, and isolate problematic changes.
In the end, mastering your equipment signifies heading outside of default configurations and shortcuts — it’s about acquiring an personal expertise in your enhancement environment to ensure that when problems come up, you’re not misplaced at midnight. The better you understand your resources, the more time you are able to invest solving the particular trouble rather then fumbling as a result of the procedure.
Reproduce the situation
Among the most essential — and sometimes disregarded — actions in efficient debugging is reproducing the problem. Prior to jumping into your code or building guesses, developers require to create a dependable natural environment or circumstance exactly where the bug reliably seems. Devoid of reproducibility, repairing a bug gets to be a game of probability, typically leading to squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting just as much context as is possible. Request questions like: What steps led to the issue? Which environment was it in — progress, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you've got, the easier it will become to isolate the exact ailments below which the bug takes place.
As soon as you’ve collected ample info, endeavor to recreate the trouble in your neighborhood atmosphere. This may imply inputting the exact same information, simulating very similar user interactions, or mimicking technique states. If The difficulty appears intermittently, look at writing automated assessments that replicate the sting circumstances or condition transitions involved. These exams not simply help expose the trouble but will also stop regressions Sooner or later.
In some cases, the issue could be natural environment-specific — it might come about only on sure operating techniques, browsers, or underneath individual configurations. Utilizing equipment like Digital devices, containerization (e.g., Docker), or cross-browser tests platforms is usually instrumental in replicating this kind of bugs.
Reproducing the trouble isn’t merely a action — it’s a mentality. It requires patience, observation, as well as a methodical technique. But when you finally can continuously recreate the bug, you might be already halfway to fixing it. With a reproducible scenario, You should use your debugging resources a lot more effectively, test possible fixes safely, and communicate much more clearly with your team or users. It turns an summary criticism right into a concrete problem — and that’s exactly where developers prosper.
Examine and Fully grasp the Mistake Messages
Error messages are frequently the most respected clues a developer has when some thing goes wrong. Rather than looking at them as discouraging interruptions, developers ought to discover to take care of mistake messages as direct communications in the system. They normally inform you just what happened, where by it took place, and at times even why it happened — if you know the way to interpret them.
Start off by studying the information meticulously and in comprehensive. Quite a few developers, specially when beneath time pressure, look at the initial line and immediately start out producing assumptions. But further while in the error stack or logs may well lie the accurate root induce. Don’t just copy and paste mistake messages into search engines like google and yahoo — go through and understand them 1st.
Break the error down into parts. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a specific file and line range? What module or function activated it? These questions can information your investigation and point you towards the responsible code.
It’s also valuable to understand the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally adhere to predictable designs, and Discovering to recognize these can substantially increase your debugging procedure.
Some glitches are imprecise or generic, and in Individuals scenarios, it’s crucial to examine the context where the mistake occurred. Examine linked log entries, enter values, and up to date modifications while in the codebase.
Don’t ignore compiler or linter warnings possibly. These frequently precede more substantial difficulties and supply hints about potential bugs.
In the end, error messages will not be your enemies—they’re your guides. Discovering to interpret them correctly turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a extra efficient and confident developer.
Use Logging Wisely
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When utilised proficiently, it offers authentic-time insights into how an software behaves, supporting you fully grasp what’s occurring beneath the hood while not having to pause execution or phase throughout the code line by line.
An excellent logging method begins with realizing what to log and at what degree. Typical logging levels include DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for comprehensive diagnostic info during development, Facts for normal functions (like productive begin-ups), WARN for probable difficulties that don’t split the application, Mistake for true difficulties, and Deadly when the procedure can’t continue on.
Keep away from flooding your logs with extreme or irrelevant data. Far too much logging can obscure critical messages and slow down your procedure. Target crucial events, condition adjustments, enter/output values, and demanding decision details within your code.
Structure your log messages Obviously and consistently. Include things like context, including timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to keep track of how variables evolve, what problems are met, and what branches of logic are executed—all with no halting This system. They’re Specifically important in manufacturing environments where by stepping by means of code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
Finally, sensible logging is about harmony and clarity. Which has a effectively-considered-out logging approach, you'll be able to lessen the time it takes to spot troubles, attain deeper visibility into your programs, and Enhance the In general maintainability and reliability of one's code.
Consider Similar to a Detective
Debugging is not just a specialized process—it is a method of investigation. To successfully recognize and deal with bugs, builders must method the method just like a detective fixing a thriller. This way of thinking allows break down complicated concerns into manageable areas and observe clues logically to uncover the foundation induce.
Start by gathering proof. Think about the symptoms of the issue: error messages, incorrect output, or overall performance concerns. Similar to a detective surveys against the law scene, accumulate just as much suitable facts as you could without the need of leaping to conclusions. Use logs, take a look at scenarios, and consumer stories to piece collectively a clear image of what’s happening.
Next, variety hypotheses. Talk to you: What may very well be triggering this behavior? Have any modifications not too long ago been created for the codebase? Has this problem occurred before less than very similar conditions? The aim is always to narrow down alternatives and establish likely culprits.
Then, examination your theories systematically. Attempt to recreate the problem inside of a managed surroundings. In the event you suspect a certain perform or element, isolate it and validate if The problem persists. Like a detective conducting interviews, talk to your code inquiries and Allow the effects direct you closer to the reality.
Spend shut consideration to little aspects. Bugs typically hide from the least envisioned areas—similar to a missing semicolon, an off-by-one error, or a race issue. Be thorough and client, resisting the urge to patch the issue with no totally knowledge it. Short-term fixes may well hide the true trouble, only for it to resurface later on.
Lastly, hold notes on what you experimented with and acquired. Just as detectives log their investigations, documenting your debugging approach can save time for potential challenges and assist Other folks understand your reasoning.
By pondering similar to a detective, builders can sharpen their analytical abilities, technique complications methodically, and turn out to be simpler at uncovering concealed challenges in complicated techniques.
Produce Checks
Writing exams is one of the best tips on how to improve your debugging abilities and Total progress performance. Checks not only assist catch bugs early but in addition function a security Internet that provides you self esteem when earning changes for your codebase. A effectively-examined application is easier to debug since it permits you to pinpoint just the place and when a challenge happens.
Begin with unit exams, which give attention to personal features or modules. These tiny, isolated exams can rapidly reveal regardless of whether a particular piece of logic is working as envisioned. Any time a take a look at fails, you promptly know the place to seem, drastically lowering the time spent debugging. Device assessments are Specifically beneficial for catching regression bugs—problems that reappear after Beforehand currently being mounted.
Subsequent, combine integration assessments and stop-to-finish checks into your workflow. These enable be certain that numerous parts of your software perform together efficiently. They’re specifically helpful for catching bugs that manifest in intricate methods with various elements or solutions interacting. If a little something breaks, your assessments can tell you which Component of the pipeline failed and underneath what situations.
Crafting exams also forces you to definitely Feel critically regarding your code. To test a aspect appropriately, you'll need to be aware of its inputs, anticipated outputs, and edge scenarios. This degree of knowledge Obviously prospects to higher code structure and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug can be a strong starting point. Once the examination fails continuously, you'll be able to center on fixing the bug and observe your take a look at pass when the issue is solved. This solution ensures that precisely the same bug doesn’t return Down the road.
In short, composing assessments turns debugging from the frustrating guessing recreation right into a structured and predictable course of action—helping you catch a lot more bugs, speedier plus more reliably.
Consider Breaks
When debugging a tricky situation, it’s uncomplicated to be immersed in the problem—staring at your display for hrs, striving Option just after solution. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lessen annoyance, and infrequently see The difficulty from the new point of view.
When you are way too near to the code for also prolonged, cognitive tiredness sets in. You could commence overlooking clear problems or misreading code which you wrote just hours earlier. Within this state, your Mind will become a lot less successful at dilemma-fixing. A short wander, a espresso split, and even switching to a special task for ten–quarter-hour can refresh your emphasis. Several developers report getting the foundation of a difficulty after they've taken time to disconnect, permitting their subconscious operate inside the background.
Breaks also assistance protect against burnout, Specially in the course of longer debugging classes. Sitting in front of a display screen, mentally stuck, is don't just unproductive and also draining. Stepping away allows you to return with renewed Electricity as well as a clearer mindset. You would possibly out of the blue notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
In case you’re stuck, a fantastic rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then have a five–10 moment break. Use that point to move all around, extend, or do anything unrelated to code. It may come to feel counterintuitive, especially underneath tight deadlines, nonetheless it actually contributes to faster and simpler debugging Ultimately.
In brief, taking breaks is just not an indication of weakness—it’s a wise system. It gives your Mind House to breathe, improves your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Every bug you experience is much more than simply A short lived setback—It is a chance to improve to be a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural difficulty, each one can teach you one thing worthwhile when you take the time to reflect and evaluate what went Improper.
Start off by inquiring on your own a handful of key concerns after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with greater techniques like device screening, code testimonials, or logging? The solutions typically reveal blind spots within your workflow or knowing and allow you to Create more powerful coding behavior relocating forward.
Documenting bugs can also be a great habit. Keep a developer journal or maintain a log in which Gustavo Woltmann AI you Observe down bugs you’ve encountered, the way you solved them, and Whatever you realized. As time passes, you’ll start to see styles—recurring difficulties or widespread blunders—which you can proactively steer clear of.
In team environments, sharing Anything you've figured out from a bug together with your friends is often Specially effective. Whether or not it’s via a Slack concept, a short generate-up, or A fast understanding-sharing session, encouraging Some others stay away from the same challenge boosts crew efficiency and cultivates a more robust Understanding society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as crucial aspects of your growth journey. In the end, a lot of the greatest builders usually are not those who compose fantastic code, but people who consistently find out from their issues.
Ultimately, Each individual bug you resolve provides a brand new layer to the talent set. So upcoming time you squash a bug, have a second to mirror—you’ll occur away a smarter, far more able developer due to it.
Summary
Improving upon your debugging expertise can take time, practice, and persistence — although the payoff is large. It tends to make you a far more economical, confident, and capable developer. The following time you happen to be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be improved at Everything you do.